- An array is a collection of data items, all of the same type, accessed using a common name.
- A one-dimensional array is like a list(vector); A two dimensional array is like a table(matrix). We can have more dimensions.
- Always, Contiguous (adjacent) memory locations are used to store array elements in memory.
- Elements of the array can be randomly accessed since we can calculate the address of each element of the array with the given base address and the size of the data element.
Array variables are declared identically to variables of their data type, except that the variable name is followed by one pair of square [ ] brackets for mentioning dimension of the array.
Dimensions used when declaring arrays in C must be positive integral constants or constant expressions.
In C99, dimensions must still be positive integers, but variables can be used, so long as the variable has a positive value at the time the array is declared. ( Space is allocated only once, at the time the array is declared. The array does NOT change sizes later if the variable used to declare it changes. )
Examples:int A[ 10 ];
float F[ 1000 ];
char S[100];
const int N = 100;
int list[N];
C99 Only Example:
int n;
printf( "How big an array do you want? " );
scanf( "%d", &n );
if( n <= 0 ) {
printf( "Error - Quitting\n");
exit( 0 );
}
double data[ n ];//This only works in C99, not in plain C
printf( "How big an array do you want? " );
scanf( "%d", &n );
if( n <= 0 ) {
printf( "Error - Quitting\n");
exit( 0 );
}
double data[ n ];//This only works in C99, not in plain C
Initializing Arrays
Arrays may be initialized when they are declared, just as any other variables.
Place the initialization data in curly {} braces following the equals sign. Note the use of commas in the examples below.
Place the initialization data in curly {} braces following the equals sign. Note the use of commas in the examples below.
An array may be partially initialized, by providing fewer data items than the size of the array. The remaining array elements will be automatically initialized to zero.
If an array is to be completely initialized, the dimension of the array is not required. The compiler will automatically size the array to fit the initialized data. ( Variation: Multidimensional arrays - see below. )
If an array is to be completely initialized, the dimension of the array is not required. The compiler will automatically size the array to fit the initialized data. ( Variation: Multidimensional arrays - see below. )
Examples:
int A[ 6 ]={ 1, 2, 3, 4, 5, 6 };
float F[100]={ 1.0f, 5.0f, 20.0f };
double F[ ]={3.141592654, 1.570796327, 0.785398163}
int A[ 6 ]={ 1, 2, 3, 4, 5, 6 };
float F[100]={ 1.0f, 5.0f, 20.0f };
double F[ ]={3.141592654, 1.570796327, 0.785398163}
Note that the array size can be avoided while initializing single dimensional array.
We can also initialize element at a particular location by using the array index
A[10]=100; will initialize 11 th element in the array to 100.Note array indexing starts from 0.
We can also initialize an array after declaration.
Designated Initializers: In C99 there is an alternate mechanism, that allows you to initialize specific elements, not necessarily at the beginning.
This method can be mixed in with traditional initialization
For example:
int numbers[ 100 ] = { 1, 2, 3, [10] = 10, 11, 12, [60] = 50, [42] = 420 };
In this example,the first three elements are initialized to 1, 2, and 3 respectively.
Then element 10 ( the 11th element ) is initialized to 10
The next two elements ( 12th and 13th ) are initialized to 11 and 12 respectively.
Element number 60 ( the 61st ) is initialized to 50, and number 42 ( the 43rd ) to 420.
This method can be mixed in with traditional initialization
For example:
int numbers[ 100 ] = { 1, 2, 3, [10] = 10, 11, 12, [60] = 50, [42] = 420 };
In this example,the first three elements are initialized to 1, 2, and 3 respectively.
Then element 10 ( the 11th element ) is initialized to 10
The next two elements ( 12th and 13th ) are initialized to 11 and 12 respectively.
Element number 60 ( the 61st ) is initialized to 50, and number 42 ( the 43rd ) to 420.
( Note that the designated initializers do not need to appear in order. )
As with traditional methods, all uninitialized values are set to zero.
If the size of the array is not given, then the largest initialized position determines the size of the array.
As with traditional methods, all uninitialized values are set to zero.
If the size of the array is not given, then the largest initialized position determines the size of the array.
Using Arrays
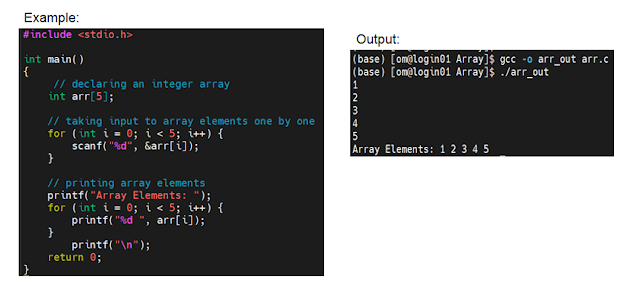
Advantages of an Array in C:
Disadvantages of an Array in C/C++:
The first sample program uses loops and arrays to calculate the first twenty Fibonacci numbers.
/* Program to calculate the first 20 Fibonacci numbers. */
#include <stdlib.h>
#include <stdio.h>
int main( void )
{
int i, fibonacci[ 20 ];
fibonacci[ 0 ] = 0;
fibonacci[ 1 ] = 1;
for( i = 2; i < 20; i++ )
fibonacci[ i ] = fibonacci[ i - 2 ] + fibonacci[ i - 1 ];
for( i = 0; i < 20; i++ )
printf( "Fibonacci[ %d ] = %f\n", i, fibonacci[ i ] );
} /* End of sample program to calculate Fibonacci numbers */
Elements of an array are accessed by specifying the index ( offset ) of the desired element within square [ ] brackets after the array name.
Array subscripts must be of integer type. ( int, long int, char, etc. )
Array subscripts must be of integer type. ( int, long int, char, etc. )
VERY IMPORTANT: Array indices start at zero in C, and go to one less than the size of the array. For example, a five element array will have indices zero through four. This is because the index in C is actually an offset from the beginning of the array. ( The first element is at the beginning of the array, and hence has zero offset. )
Landmine: The most common mistake when working with arrays in C is forgetting that indices start at zero and stop one less than the array size.
Arrays are commonly used in conjunction with loops, in order to perform the same calculations on all ( or some part ) of the data items in the array. Eg:A[0]=12; will store 12 in the array 0th location //updating array using index
printf("%d",A[2]) will print the 3 rd element in the array.// accessing the array element using index
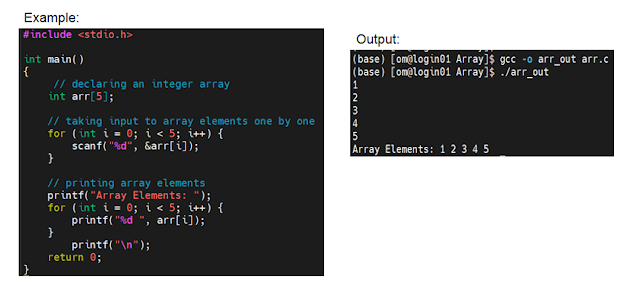
Advantages of an Array in C:
- Random access of elements using array index.
- Use of less line of code as it creates a single array of multiple elements.
- Easy access to all the elements.
- Traversal through the array becomes easy using a single loop.
- Searching and Sorting becomes easy as it can be accomplished by writing less line of code.
Disadvantages of an Array in C/C++:
- Allows a fixed number of elements to be entered which is decided at the time of declaration. Unlike a linked list, an array in C is not dynamic.
- Insertion and deletion of elements can be costly since the elements are needed to be managed in accordance with the new memory allocation.
Sample Programs Using 1-D Arrays
/* Program to calculate the first 20 Fibonacci numbers. */
#include <stdlib.h>
#include <stdio.h>
int main( void )
{
int i, fibonacci[ 20 ];
fibonacci[ 0 ] = 0;
fibonacci[ 1 ] = 1;
for( i = 2; i < 20; i++ )
fibonacci[ i ] = fibonacci[ i - 2 ] + fibonacci[ i - 1 ];
for( i = 0; i < 20; i++ )
printf( "Fibonacci[ %d ] = %f\n", i, fibonacci[ i ] );
} /* End of sample program to calculate Fibonacci numbers */
Program to read n numbers and find the largest using array(university question)
#include <stdlib.h>
#include <stdio.h>
int main( void )
{
int i, n,a[100],large;
printf("enter...n\n”);
scanf(“%d”,&n);
printf(“Enter the elements\n”);
for( i = 0; i < n; i++ )
scanf(“%d”,&a[i]);
large=a[0];
f or( i = 1; i < n; i++ )
if (a[i]>large) large=a[i]
printf( "Largest Element in the array is %d”,large);
}
Write a C program to find second largest element in an array(university qn)
#include <stdlib.h>
#include <stdio.h>
int main( void )
{
int i, n,a[100],large,slarge=-1;
printf("enter...n\n");
scanf("%d",&n);
printf("Enter the elements\n");
for( i = 0; i < n; i++ )
scanf("%d",&a[i]);
large=a[0];
for( i = 1; i < n; i++ )
if (a[i]>large) {slarge=large;large=a[i];}
else if (a[i]>slarge) slarge=a[i];
printf( "Largest Element in the array is %d ",large);
if(slarge==-1)
printf("No second largest\n");
else
printf(" second largest is =%d",slarge);
}
#include <stdio.h>
int main()
{
int a[100],b[100],c[100],i,j,k,n,m;
printf("Enter the number of elements in array a:");
scanf("%d",&n);
printf("Enter the array elements in sorted order....\n");
for(i=0;i<n;i++)
scanf("%d",&a[i]);
printf("Enter the number of elements in array b:");
scanf("%d",&m);
printf("Enter the array elements in sorted order....\n");
for(i=0;i<m;i++)
scanf("%d",&b[i]);
for(i=0,j=0,k=0;i<n&&j<m;k++)
{
if(a[i]<b[j])
c[k]=a[i++];
else
c[k]=b[j++];
}
while(i<n) c[k++]=a[i++];
while(j<m) c[k++]=b[j++];
printf("The merged array is...\n");
for(i=0;i<k;i++)
printf("%d\n",c[i]);
}
Write a C program to find the occurrence of each element in an array.(uq)
#include <stdio.h> int main()
{
//Initialize array
int arr[50];
int n,i,j,count;
//Array fr will store frequencies of element
int fr[50];
int visited = -1; // -1 indcated element already considered
printf("Enter n \n");
scanf("%d",&n);
printf("Enter elements\n");
for(i=0;i<n;i++)
scanf("%d",&arr[i]);
for( i = 0; i < n; i++){
count = 1;
for(j = i+1; j < n; j++)
{
if(arr[i] == arr[j]){
count++;
//To avoid counting same element again
fr[j] = visited;
}
}
if(fr[i] != visited)
fr[i] = count;
}
//Displays the frequency of each element present in array
printf("---------------------\n");
printf(" Element | Frequency\n");
printf("---------------------\n");
for(int i = 0; i < n; i++){
if(fr[i] != visited){
printf("%5d", arr[i]);
printf(" | ");
printf("%5d\n", fr[i]);
}
}
printf("---------------------\n");
return 0;
}
Linear Search
#include <stdio.h>void main()
{
int arr[50],key,i,n,flag=0;
printf("Enter the number of elements n<=50\n");
scanf("%d",&n);
printf("Enter %d elements\n",n);
for(i=0;i<n;i++)
{
scanf("%d",&arr[i]);
}
printf("Enter a key element to search\n");
scanf("%d",&key);
for(i=0;i<n;i++)
{
if(arr[i]==key)
{
flag=1;
break;
}
}
if(flag==1)
printf("%d is found in the list, at position %d\n",key,i+1);
else
printf("%d is not in the list\n",key);
}
Write a C program to check if a number is present in a given list of numbers. If present, give location of the number otherwise insert the number in the list at the end.(uq)
#include <stdio.h>
main()
{
int arr[51],k,i,n,flag=0;
printf("Enter the number of elements n<50\n");
scanf("%d",&n);
printf("Enter %d elements\n",n);
for(i=0;i<n;i++)
{
scanf("%d",&arr[i]);
}
printf("Enter a number to be search\n");
scanf("%d",&k);
for(i=0;i<n;i++)
{
if(arr[i]==k)
{
flag=1;
break;
}
}
if(flag==1)
printf("%d is found in the list, at position %d\n",k,i+1);
else
{printf("%d is not in the list\n",k);
printf("element is inserted at end...\n");
arr[n]=k;
for(i=0;i<=n;i++)
printf("%3d",arr[i]);
}
}
#include <stdio.h>
main()
{
int arr[51],k,i,n,flag=0;
printf("Enter the number of elements n<50\n");
scanf("%d",&n);
printf("Enter %d elements\n",n);
for(i=0;i<n;i++)
{
scanf("%d",&arr[i]);
}
printf("Enter a number to be search\n");
scanf("%d",&k);
for(i=0;i<n;i++)
{
if(arr[i]==k)
{
flag=1;
break;
}
}
if(flag==1)
printf("%d is found in the list, at position %d\n",k,i+1);
else
{printf("%d is not in the list\n",k);
printf("element is inserted at end...\n");
arr[n]=k;
for(i=0;i<=n;i++)
printf("%3d",arr[i]);
}
}
Bubble sort
#include <stdio.h>void main()
{
int arr[50],i,j,n,exchng,temp;
printf("Enter the number of elements n<=50\n");
scanf("%d",&n);
printf("Enter %d elements\n",n);
for(i=0;i<n;i++)
scanf("%d",&arr[i]);
for(i=0;i<n-1;i++)
{exchng=0;
for(j=0;j<n-1-i;j++)
{
if (arr[j]>arr[j+1])
{
temp=arr[j];
arr[j]=arr[j+1];
arr[j+1]=temp;
exchng=1;
}
}
if(exchng==0) break;
}
printf("Sorted List...\n");
for(i=0;i<n;i++)
printf("%d\n",arr[i]);
}
Finding the unique and duplicate element in array
#include <stdio.h>
void main()
{
int arr[50],i,j,n,exchng,temp,c;
printf("Enter the number of elements n<=50\n");
scanf("%d",&n);
printf("Enter %d elements\n",n);
for(i=0;i<n;i++)
scanf("%d",&arr[i]);
for(i=0;i<n-1;i++)
{exchng=0;
for(j=0;j<n-1-i;j++)
{
if (arr[j]>arr[j+1])
{
temp=arr[j];
arr[j]=arr[j+1];
arr[j+1]=temp;
exchng=1;
}
}
if(exchng==0) break;
}
printf("Sorted List...\n");
for(i=0;i<n;i++)
printf("%d\n",arr[i]);
// finding duplicate elements
for(i=0;i<n;i+=c)
{c=1;
for(j=i+1;j<n;j++)
if(arr[i]==arr[j]) c++;
if(c>1) printf("%d is duplicate...occures %d times\n",arr[i],c);
else printf("%d is unique\n",arr[i]);
}
}
void main()
{
int arr[50],i,j,n,exchng,temp,c;
printf("Enter the number of elements n<=50\n");
scanf("%d",&n);
printf("Enter %d elements\n",n);
for(i=0;i<n;i++)
scanf("%d",&arr[i]);
for(i=0;i<n-1;i++)
{exchng=0;
for(j=0;j<n-1-i;j++)
{
if (arr[j]>arr[j+1])
{
temp=arr[j];
arr[j]=arr[j+1];
arr[j+1]=temp;
exchng=1;
}
}
if(exchng==0) break;
}
printf("Sorted List...\n");
for(i=0;i<n;i++)
printf("%d\n",arr[i]);
// finding duplicate elements
for(i=0;i<n;i+=c)
{c=1;
for(j=i+1;j<n;j++)
if(arr[i]==arr[j]) c++;
if(c>1) printf("%d is duplicate...occures %d times\n",arr[i],c);
else printf("%d is unique\n",arr[i]);
}
}
Multidimensional Arrays
- Multi-dimensional arrays are declared by providing more than
one set of square [ ] brackets after the variable name in the
declaration statement.
- One dimensional arrays do not require the dimension to be
given if the array is to be completely initialized. By
analogy, multi-dimensional arrays do not require the first
dimension to be given if the array is to be completely initialized.
All dimensions after the first must be given in any case.
- For two dimensional arrays, the first dimension is commonly
considered to be the number of rows, and the second dimension the
number of columns.
- Two dimensional arrays are considered by C/C++ to be an array
of (single dimensional arrays ). For example, "int
numbers[ 5 ][ 6 ]" would refer to a single dimensional
array of 5 elements, wherein each element is a single dimensional
array of 6 integers. By extension, "int numbers[ 12 ][ 5 ][ 6
]" would refer to an array of twelve elements, each of which is
a two dimensional array, and so on.
- Another way of looking at this is that C stores two
dimensional arrays by rows, with all elements of a row being stored
together as a single unit. Knowing this can sometimes lead to
more efficient programs.
- Multidimensional arrays may be completely initialized by
listing all data elements within a single pair of curly {} braces,
as with single dimensional arrays.
- It is better programming practice to enclose each row within
a separate subset of curly {} braces, to make the program more
readable. This is required if any row other than the last is
to be partially initialized. When subsets of braces are used,
the last item within braces is not followed by a comma, but the
subsets are themselves separated by commas.
- Multidimensional arrays may be partially initialized by not
providing complete initialization data. Individual rows of a
multidimensional array may be partially initialized, provided that
subset braces are used.
- Individual data items in a multidimensional array are
accessed by fully qualifying an array element. Alternatively,
a smaller dimensional array may be accessed by partially qualifying
the array name. For example, if "data" has
been declared as a three dimensional array of floats, then
data[ 1 ][ 2 ][ 5 ] would refer to a
float, data[ 1 ][ 2 ] would refer to a
one-dimensional array of floats, and data[ 1 ] would refer
to a two-dimensional array of floats. The reasons for this and
the incentive to do this relate to memory-management issues that are
beyond the scope of these notes.
Example declaring and initializing 2 dimensional arrays
The two-dimensional array can be defined as an array of arrays. The 2D array is organized as matrices which can be represented as the collection of rows and columns.
Declaration
int A[ 3 ][ 5 ]; /* 3 rowsby 5 columns */
int A[ 3 ][ 5 ]; /* 3 rowsby 5 columns */
const int NROWS=10;
const int NCOLS = 20;
float matrix[ NROWS ][ NCOLS ];
const int NCOLS = 20;
float matrix[ NROWS ][ NCOLS ];
In the 1D array, we don't need to specify the size of the array if the declaration and initialization are being done simultaneously. However, this will not work with 2D arrays. We will have to define at least the second dimension of the array.
Initialization
int arr[2][3]={{1,2,3},{4,5,6}}; int arr[2][3]={1,2,3,4,5,6};
int arr[][3]={1,2,3,4,5,6};
The following initialization are Invalid
int arr[][]={1,2,3,4,5,6};
int arr[3][]={1,2,3,4,5,6};
Accessing elements
Elements are accessed using both the row and column index in 2D arrays
arr[2][1]=10 will store element in third row second column.( Indexing start with 0)
printf("%d",arr[2][1]) will print the same.
Lets consider a 2D array abc[5][4]. The conceptual memory representation is shown below.
Row major and column major order representation
In computing, row-major order and column-major order are methods for storing multidimensional arrays in linear storage such as random access memory.
The difference between the orders lies in which elements of an array are contiguous in memory. In row-major order, the consecutive elements of a row reside next to each other, whereas the same holds true for consecutive elements of a column in column-major order.
The difference between the orders lies in which elements of an array are contiguous in memory. In row-major order, the consecutive elements of a row reside next to each other, whereas the same holds true for consecutive elements of a column in column-major order.
Example
Sample Program Using 2-D Arrays
Sum of the elements in each row of a matrix
#include <stdio.h>
void main()
{
int A[50][50],B[50][50],rsum;
int r,c,m,n;
printf("Enter the size of the matrix nof of rows,columns\n");
scanf("%d%d",&m,&n);
printf("Enter the elements of matrix A row by row\n");
for(r=0;r<m;r++)
for(c=0;c<n;c++)
scanf("%d",&A[r][c]);
//computing transpose
for(r=0;r<m;r++)
{rsum=0;
for(c=0;c<n;c++)
rsum=rsum+A[r][c];
printf("Sum of elements in row %d=%d\n",r,rsum);
}
}
void main()
{
int A[50][50],B[50][50],rsum;
int r,c,m,n;
printf("Enter the size of the matrix nof of rows,columns\n");
scanf("%d%d",&m,&n);
printf("Enter the elements of matrix A row by row\n");
for(r=0;r<m;r++)
for(c=0;c<n;c++)
scanf("%d",&A[r][c]);
//computing transpose
for(r=0;r<m;r++)
{rsum=0;
for(c=0;c<n;c++)
rsum=rsum+A[r][c];
printf("Sum of elements in row %d=%d\n",r,rsum);
}
}
Transpose of a matrix A^T=B ( university question)
#include <stdio.h>
main()
{
int A[50][50],B[50][50];
int r,c,m,n;
printf("Enter the size of the matrix nof of rows,columns\n");
scanf("%d%d",&m,&n);
printf("Enter the elements of matrix A row by row\n");
for(r=0;r<m;r++)
for(c=0;c<n;c++)
scanf("%d",&A[r][c]);
//computing transpose
for(r=0;r<m;r++)
for(c=0;c<n;c++)
B[c][r]=A[r][c];
printf("The transpose of A is \n");
for(r=0;r<n;r++)
{
for(c=0;c<m;c++)
printf("%3d",B[r][c]);
printf("\n");
}
}
Adding two matrix sum=a+b ( university question)
#include <stdlib.h>
#include <stdio.h>
int main( void )
{
int a[ 2 ][ 3 ] = { { 5, 6, 7 }, { 10, 20, 30 } };
int b[ 2 ][ 3 ] = { { 1, 2, 3 }, { 3, 2, 1 } };
int sum[ 2 ][ 3 ], row, column;
/* First the addition */
for( row = 0; row < 2; row++ )
for( column = 0; column < 3; column++ )
sum[ row ][ column ] =a[ row ][ column ] + b[ row ][ column ];
/* Then print the results */
printf( "The sum is: \n\n" );
for( row = 0; row < 2; row++ ) {
for( column = 0; column < 3; column++ )
printf( "\t%d", sum[ row ][ column ] );
printf( '\n' ); /* at end of each row */
}
return 0;
}
Multiply two matrix C=A*B ( university question)
#include<stdio.h>
int main(void)
{
int i,j,k,m,n,p,q,tot;
int A[30][30], B[30][30], C[30][30];
printf(" Please insert the number of rows and columns for first matrix \n ");
scanf("%d%d", &m, &n);
printf(" Insert your matrix elements : \n ");
for (i= 0; i < m; i++)
for (j = 0; j < n; j++)
scanf("%d", &A[i][j]);
printf("Please insert the number of rows and columns for second matrix\n");
scanf("%d%d", &p, &q);
if (n != p)
printf(" Your given matrices cannot be multiplied with each other. \n ");
else
{
printf(" Insert your elements for second matrix \n ");
for (i= 0; i< p; i++)
for (j= 0; j< q; j++)
scanf("%d", &B[i][j] );
for (i = 0; i < m; i++) {
for (j = 0; j < q; j++) {
#include <stdio.h>
main()
{
int A[50][50],B[50][50];
int r,c,m,n;
printf("Enter the size of the matrix nof of rows,columns\n");
scanf("%d%d",&m,&n);
printf("Enter the elements of matrix A row by row\n");
for(r=0;r<m;r++)
for(c=0;c<n;c++)
scanf("%d",&A[r][c]);
//computing transpose
for(r=0;r<m;r++)
for(c=0;c<n;c++)
B[c][r]=A[r][c];
printf("The transpose of A is \n");
for(r=0;r<n;r++)
{
for(c=0;c<m;c++)
printf("%3d",B[r][c]);
printf("\n");
}
}
Adding two matrix sum=a+b ( university question)
#include <stdlib.h>
#include <stdio.h>
int main( void )
{
int a[ 2 ][ 3 ] = { { 5, 6, 7 }, { 10, 20, 30 } };
int b[ 2 ][ 3 ] = { { 1, 2, 3 }, { 3, 2, 1 } };
int sum[ 2 ][ 3 ], row, column;
/* First the addition */
for( row = 0; row < 2; row++ )
for( column = 0; column < 3; column++ )
sum[ row ][ column ] =a[ row ][ column ] + b[ row ][ column ];
/* Then print the results */
printf( "The sum is: \n\n" );
for( row = 0; row < 2; row++ ) {
for( column = 0; column < 3; column++ )
printf( "\t%d", sum[ row ][ column ] );
printf( '\n' ); /* at end of each row */
}
return 0;
}
Multiply two matrix C=A*B ( university question)
#include<stdio.h>
int main(void)
{
int i,j,k,m,n,p,q,tot;
int A[30][30], B[30][30], C[30][30];
printf(" Please insert the number of rows and columns for first matrix \n ");
scanf("%d%d", &m, &n);
printf(" Insert your matrix elements : \n ");
for (i= 0; i < m; i++)
for (j = 0; j < n; j++)
scanf("%d", &A[i][j]);
printf("Please insert the number of rows and columns for second matrix\n");
scanf("%d%d", &p, &q);
if (n != p)
printf(" Your given matrices cannot be multiplied with each other. \n ");
else
{
printf(" Insert your elements for second matrix \n ");
for (i= 0; i< p; i++)
for (j= 0; j< q; j++)
scanf("%d", &B[i][j] );
for (i = 0; i < m; i++) {
for (j = 0; j < q; j++) {
tot=0;
for (k = 0; k < p; k++) {
tot = tot + A[i][k] * B[k][j];
}
C[i][j] = tot;
}
}
printf(" The result of matrix multiplication or product of the matrices is: \n ");
for (i = 0; i < m; i++) {
for (j = 0;j < q; j++)
printf("%5d", C[i][j] );
printf(" \n ");
}
}
return 0;
}
for (k = 0; k < p; k++) {
tot = tot + A[i][k] * B[k][j];
}
C[i][j] = tot;
}
}
printf(" The result of matrix multiplication or product of the matrices is: \n ");
for (i = 0; i < m; i++) {
for (j = 0;j < q; j++)
printf("%5d", C[i][j] );
printf(" \n ");
}
}
return 0;
}
Check whether the given matrix is diagonal ( university question)
#include <stdio.h>
int main(void)
{
int i,j,m,n,flag=1;
int A[30][30];
printf(" Please insert the number of rows and columns max 30 \n ");
scanf("%d%d", &m, &n);
printf(" Insert your matrix elements : \n ");
for (i= 0; i < m; i++)
for (j = 0; j < n; j++)
scanf("%d", &A[i][j]);
//checking for non diagonal ..all elements must be 0
for (i= 0; i < m; i++)
{
for (j = 0; j < n; j++)
if(i!=j && A[i][j]!=0) {flag=0; break;}
if(flag==0) break;
}
if(flag)
printf("Diagonal Matrix \n");
else
printf("Non Diagonal Matrix\n");
}
Write a C program to check whether a matrix is symmetric or skew symmetric
#include <stdio.h>
#define MAX_SIZE 10
int main() {
int A[MAX_SIZE][MAX_SIZE];
int i, j, rows, cols;
int symmetric = 1, skewsymmetric = 1;
printf("Enter the number of rows and columns of the square matrix: ");
scanf("%d", &rows);
cols = rows;
printf("Enter the elements of the matrix:\n");
for (i = 0; i < rows; i++) {
for (j = 0; j < cols; j++) {
scanf("%d", &A[i][j]);
}
}
// Check for symmetric matrix
for (i = 0; i < rows; i++) {
for (j = 0; j < cols; j++) {
if (A[i][j] != A[j][i]) {
symmetric = 0;
break;
}
}
if (symmetric == 0) {
break;
}
}
// Check for skew-symmetric matrix
for (i = 0; i < rows; i++) {
for (j = 0; j < cols; j++) {
if (A[i][j] != -A[j][i]) {
skewsymmetric = 0;
break;
}
}
if (skewsymmetric == 0) {
break;
}
}
if (symmetric == 1) {
printf("The matrix is symmetric.\n");
} else if (skewsymmetric == 1) {
printf("The matrix is skew-symmetric.\n");
} else {
printf("The matrix is neither symmetric nor skew-symmetric.\n");
}
return 0;
}
#define MAX_SIZE 10
int main() {
int A[MAX_SIZE][MAX_SIZE];
int i, j, rows, cols;
int symmetric = 1, skewsymmetric = 1;
printf("Enter the number of rows and columns of the square matrix: ");
scanf("%d", &rows);
cols = rows;
printf("Enter the elements of the matrix:\n");
for (i = 0; i < rows; i++) {
for (j = 0; j < cols; j++) {
scanf("%d", &A[i][j]);
}
}
// Check for symmetric matrix
for (i = 0; i < rows; i++) {
for (j = 0; j < cols; j++) {
if (A[i][j] != A[j][i]) {
symmetric = 0;
break;
}
}
if (symmetric == 0) {
break;
}
}
// Check for skew-symmetric matrix
for (i = 0; i < rows; i++) {
for (j = 0; j < cols; j++) {
if (A[i][j] != -A[j][i]) {
skewsymmetric = 0;
break;
}
}
if (skewsymmetric == 0) {
break;
}
}
if (symmetric == 1) {
printf("The matrix is symmetric.\n");
} else if (skewsymmetric == 1) {
printf("The matrix is skew-symmetric.\n");
} else {
printf("The matrix is neither symmetric nor skew-symmetric.\n");
}
return 0;
}
Note: Matrix programs can be written using functions and hence optimize the code.Look at the blog post on functions.
Programs to try using 1-D arrays
1.Read an array and rotate elements right( read n-number of times to rotate)
2.Find the average of list of numbers.
3.Find the maximum and minimum values from an array of integers.
4.Swap the k th and k+1 th element in an array. Read k.
5.Find the binary equivalent of a number using array.
6.Find similar elements in an array and compute the number of times they occur.
7. Separate odd and even integers in separate arrays from a given array.
8.Find the intersection of two set of numbers.
9.Rearrange n numbers in an array in reverse order.(selection sort)
10.Sort the numbers stored in array in ascending order.(bubble sort)
11.Arrange numbers in an array in such a way that the array will have odd numbers followed by the even numbers.
12.Find the frequency of digits in a set of numbers.
13.Remove duplicate elements from an array.( Hint: sort the array)
14.Merge two sorted arrays into another array in sorted order.
15.Find the mean,variance,median and standard deviation of set of integer data.
16.Read list of numbers and print prime numbers from the list.
17.List of internal marks of a subject is stored in an array ( marks out of 50).
2.Find the average of list of numbers.
3.Find the maximum and minimum values from an array of integers.
4.Swap the k th and k+1 th element in an array. Read k.
5.Find the binary equivalent of a number using array.
6.Find similar elements in an array and compute the number of times they occur.
7. Separate odd and even integers in separate arrays from a given array.
8.Find the intersection of two set of numbers.
9.Rearrange n numbers in an array in reverse order.(selection sort)
10.Sort the numbers stored in array in ascending order.(bubble sort)
11.Arrange numbers in an array in such a way that the array will have odd numbers followed by the even numbers.
12.Find the frequency of digits in a set of numbers.
13.Remove duplicate elements from an array.( Hint: sort the array)
14.Merge two sorted arrays into another array in sorted order.
15.Find the mean,variance,median and standard deviation of set of integer data.
16.Read list of numbers and print prime numbers from the list.
17.List of internal marks of a subject is stored in an array ( marks out of 50).
Find
a.number of passed students ( need 50 percentage or more)
b.number of failed students
c.number of students having 60 percentage or more
d.maximum , minimum and average mark in the subject.
( do it as a menu driven program..)
18.Search a key element in a given array ( linear search)
19.Search a key element in the give sorted array ( binary search)
20.Generate Fibonacci numbers up to n and store it in an array.Read and print prime numbers from it.
21.Given two vectors V1 and V2 in R3 . Find their sum (V1+V2)and dot product V1.V2.
22.Find the length of a given vector in Rn.
23.Find the second largest element in an unsorted array.
24.Find the kth largest element in a list.
a.number of passed students ( need 50 percentage or more)
b.number of failed students
c.number of students having 60 percentage or more
d.maximum , minimum and average mark in the subject.
( do it as a menu driven program..)
18.Search a key element in a given array ( linear search)
19.Search a key element in the give sorted array ( binary search)
20.Generate Fibonacci numbers up to n and store it in an array.Read and print prime numbers from it.
21.Given two vectors V1 and V2 in R3 . Find their sum (V1+V2)and dot product V1.V2.
22.Find the length of a given vector in Rn.
23.Find the second largest element in an unsorted array.
24.Find the kth largest element in a list.
25.Convert a decimal number into hexadecimal.
26.Write a C program to find the occurrence of each element in an array.(uq)
27.Write a C program to check if a number is present in a given list of numbers. If present, give location of the number otherwise insert the number in the list at the end.(uq)
28.Find the second largest element in the array ( uq)
29.Find the element which occurs largest number of times in an array.
30.Reverse an array.
Programs to try using two dimensional arrays
1.Read a 2D array and print it in row major and column major order.
2.Write programs for matrix arithmetic.
a)addition b)subtraction c)multiplication
3.Find transpose of a given matrix.(uq)
4.Find the trace of a matrix.( sum of the diagonal element)
5.Find sum of each rows and columns of a matrix.
a)addition b)subtraction c)multiplication
3.Find transpose of a given matrix.(uq)
4.Find the trace of a matrix.( sum of the diagonal element)
5.Find sum of each rows and columns of a matrix.
6.Find the norm of a matrix.(1-norm .Find sum of absolute value of element column wise.Find the max).
7.Given two square matrices A and B of same order.
Check whether AB equal BA.
8.Check whether the given matrix is symmetric.(university question)
9.Check whether a given matrix is an identity matrix.
10. Calculate determinant of a 3 x 3 matrix.
11.Find the saddle points in a matrix.
12.Check for upper triangular/lower triangular matrix.
13. marks(out of 150) of 5 students in 3 subjects are stored in matrix form.Find
a)top marks in each subject
b)Number of failed students in each subject
c)average mark in each subject.
d)Number of supply subjects each students have.
14.Print all prime numbers from a matrix.(university question)
15.Write a C program to accept a two dimensional matrix and display the row sum, column sum and diagonal sum of elements.(university question)
7.Given two square matrices A and B of same order.
Check whether AB equal BA.
8.Check whether the given matrix is symmetric.(university question)
9.Check whether a given matrix is an identity matrix.
10. Calculate determinant of a 3 x 3 matrix.
11.Find the saddle points in a matrix.
12.Check for upper triangular/lower triangular matrix.
13. marks(out of 150) of 5 students in 3 subjects are stored in matrix form.Find
a)top marks in each subject
b)Number of failed students in each subject
c)average mark in each subject.
d)Number of supply subjects each students have.
14.Print all prime numbers from a matrix.(university question)
15.Write a C program to accept a two dimensional matrix and display the row sum, column sum and diagonal sum of elements.(university question)
16.Check whether the given matrix is diagonal.
Comments
Post a Comment